commit
1b759ef066
175 changed files with 16163 additions and 0 deletions
@ -0,0 +1,114 @@ |
|||||||
|
using System; |
||||||
|
using System.Collections.Generic; |
||||||
|
using System.Linq; |
||||||
|
using System.Text; |
||||||
|
using System.Threading.Tasks; |
||||||
|
|
||||||
|
namespace UltimateBag |
||||||
|
{ |
||||||
|
|
||||||
|
/// <summary> |
||||||
|
/// Class that specifies how a setting should be displayed inside the ConfigurationManager settings window. |
||||||
|
/// |
||||||
|
/// Usage: |
||||||
|
/// This class template has to be copied inside the plugin's project and referenced by its code directly. |
||||||
|
/// make a new instance, assign any fields that you want to override, and pass it as a tag for your setting. |
||||||
|
/// |
||||||
|
/// If a field is null (default), it will be ignored and won't change how the setting is displayed. |
||||||
|
/// If a field is non-null (you assigned a value to it), it will override default behavior. |
||||||
|
/// </summary> |
||||||
|
/// |
||||||
|
/// <example> |
||||||
|
/// Here's an example of overriding order of settings and marking one of the settings as advanced: |
||||||
|
/// <code> |
||||||
|
/// // Override IsAdvanced and Order |
||||||
|
/// Config.AddSetting("X", "1", 1, new ConfigDescription("", null, new ConfigurationManagerAttributes { IsAdvanced = true, Order = 3 })); |
||||||
|
/// // Override only Order, IsAdvanced stays as the default value assigned by ConfigManager |
||||||
|
/// Config.AddSetting("X", "2", 2, new ConfigDescription("", null, new ConfigurationManagerAttributes { Order = 1 })); |
||||||
|
/// Config.AddSetting("X", "3", 3, new ConfigDescription("", null, new ConfigurationManagerAttributes { Order = 2 })); |
||||||
|
/// </code> |
||||||
|
/// </example> |
||||||
|
/// |
||||||
|
/// <remarks> |
||||||
|
/// You can read more and see examples in the readme at https://github.com/BepInEx/BepInEx.ConfigurationManager |
||||||
|
/// You can optionally remove fields that you won't use from this class, it's the same as leaving them null. |
||||||
|
/// </remarks> |
||||||
|
#pragma warning disable 0169, 0414, 0649 |
||||||
|
internal sealed class ConfigurationManagerAttributes |
||||||
|
{ |
||||||
|
/// <summary> |
||||||
|
/// Should the setting be shown as a percentage (only use with value range settings). |
||||||
|
/// </summary> |
||||||
|
public bool? ShowRangeAsPercent; |
||||||
|
|
||||||
|
/// <summary> |
||||||
|
/// Custom setting editor (OnGUI code that replaces the default editor provided by ConfigurationManager). |
||||||
|
/// See below for a deeper explanation. Using a custom drawer will cause many of the other fields to do nothing. |
||||||
|
/// </summary> |
||||||
|
public System.Action<BepInEx.Configuration.ConfigEntryBase> CustomDrawer; |
||||||
|
|
||||||
|
/// <summary> |
||||||
|
/// Show this setting in the settings screen at all? If false, don't show. |
||||||
|
/// </summary> |
||||||
|
public bool? Browsable; |
||||||
|
|
||||||
|
/// <summary> |
||||||
|
/// Category the setting is under. Null to be directly under the plugin. |
||||||
|
/// </summary> |
||||||
|
public string Category; |
||||||
|
|
||||||
|
/// <summary> |
||||||
|
/// If set, a "Default" button will be shown next to the setting to allow resetting to default. |
||||||
|
/// </summary> |
||||||
|
public object DefaultValue; |
||||||
|
|
||||||
|
/// <summary> |
||||||
|
/// Force the "Reset" button to not be displayed, even if a valid DefaultValue is available. |
||||||
|
/// </summary> |
||||||
|
public bool? HideDefaultButton; |
||||||
|
|
||||||
|
/// <summary> |
||||||
|
/// Force the setting name to not be displayed. Should only be used with a <see cref="CustomDrawer"/> to get more space. |
||||||
|
/// Can be used together with <see cref="HideDefaultButton"/> to gain even more space. |
||||||
|
/// </summary> |
||||||
|
public bool? HideSettingName; |
||||||
|
|
||||||
|
/// <summary> |
||||||
|
/// Optional description shown when hovering over the setting. |
||||||
|
/// Not recommended, provide the description when creating the setting instead. |
||||||
|
/// </summary> |
||||||
|
public string Description; |
||||||
|
|
||||||
|
/// <summary> |
||||||
|
/// Name of the setting. |
||||||
|
/// </summary> |
||||||
|
public string DispName; |
||||||
|
|
||||||
|
/// <summary> |
||||||
|
/// Order of the setting on the settings list relative to other settings in a category. |
||||||
|
/// 0 by default, higher number is higher on the list. |
||||||
|
/// </summary> |
||||||
|
public int? Order; |
||||||
|
|
||||||
|
/// <summary> |
||||||
|
/// Only show the value, don't allow editing it. |
||||||
|
/// </summary> |
||||||
|
public bool? ReadOnly; |
||||||
|
|
||||||
|
/// <summary> |
||||||
|
/// If true, don't show the setting by default. User has to turn on showing advanced settings or search for it. |
||||||
|
/// </summary> |
||||||
|
public bool? IsAdvanced; |
||||||
|
|
||||||
|
/// <summary> |
||||||
|
/// Custom converter from setting type to string for the built-in editor textboxes. |
||||||
|
/// </summary> |
||||||
|
public System.Func<object, string> ObjToStr; |
||||||
|
|
||||||
|
/// <summary> |
||||||
|
/// Custom converter from string to setting type for the built-in editor textboxes. |
||||||
|
/// </summary> |
||||||
|
public System.Func<string, object> StrToObj; |
||||||
|
} |
||||||
|
|
||||||
|
} |
@ -0,0 +1,453 @@ |
|||||||
|
using BepInEx; |
||||||
|
using BepInEx.Configuration; |
||||||
|
using HarmonyLib; |
||||||
|
using System; |
||||||
|
using UnityEngine; |
||||||
|
|
||||||
|
namespace UltimateBag |
||||||
|
{ |
||||||
|
[HarmonyPatch] |
||||||
|
[BepInPlugin(ID, NAME, VERSION)] |
||||||
|
public class UltimateBag : BaseUnityPlugin |
||||||
|
{ |
||||||
|
|
||||||
|
static public UltimateBag Instance { get; private set; } |
||||||
|
const string ID = "com.ness.UltimateBag"; |
||||||
|
const string NAME = "UltimateBag"; |
||||||
|
const string VERSION = "1.0"; |
||||||
|
|
||||||
|
|
||||||
|
internal void Awake() |
||||||
|
{ |
||||||
|
Instance = this; |
||||||
|
|
||||||
|
new Harmony("com.ness.UltimateBag").PatchAll(); |
||||||
|
|
||||||
|
Logger.LogMessage("UltimateBag is awake"); |
||||||
|
Update(); |
||||||
|
|
||||||
|
// CURRENT PREFAB |
||||||
|
UltimateBag.BagPrefabName = Config.Bind<string>("1.GENERAL", "Bag Visual", "5300120_PrimitiveSatchelBackpack_v", new ConfigDescription("Apply visual on the selected bag", new AcceptableValueList<string>( |
||||||
|
"5300000_AdventurerBackpack_v", |
||||||
|
"5300140_TraderFrameBackpack_v", |
||||||
|
"5300130_StrongBoxBackpack_v", |
||||||
|
"5300030_MasterTraderBackpack_v", |
||||||
|
"5300180_HornBackpack_v", |
||||||
|
"5300170_MageBackpack_v", |
||||||
|
"5380003_WeaverBackpack_v", |
||||||
|
"5300160_PreservationBackpack_v", |
||||||
|
"5300110_NomadBackpack_v", |
||||||
|
"5300041_ProspectorBackpackElite_v", |
||||||
|
"5300190_BoozuBackpack_v", |
||||||
|
"5300070_Wolfcase_v", |
||||||
|
"5300040_ProspectorBackpack_v", |
||||||
|
"5380002_ChalecedonyBackpack_v", |
||||||
|
"5380005_BrigandBackpack_v", |
||||||
|
"5300050_GlowstoneBackpack_v", |
||||||
|
"5380001_ChargedStoneBackpack_v", |
||||||
|
"5300120_PrimitiveSatchelBackpack_v"))); |
||||||
|
|
||||||
|
// SLOT 1 |
||||||
|
UltimateBag.EnableSlot1 = Config.Bind<bool>("2. WEAPON SLOT 1", "Enable Weapon Slot 1", true, "Add a weapon on your bag"); |
||||||
|
UltimateBag.VisualSlot1 = Config.Bind<int>("2. WEAPON SLOT 1", "Weapon ID", 2200000, "ItemID of the weapon you want to attach"); |
||||||
|
UltimateBag.PositionSlot1 = Config.Bind<string>("2. WEAPON SLOT 1", "Weapon Type", "Sword", new ConfigDescription("Choose the type of weapon select in Slot1. Required for positionning.", new AcceptableValueList<string>("Bow","Spear","Staff", "Axe(One Handed)", "Axe(Two Handed)", "Halberd", "Sword(One Handed)", "Sword(Two Handed)","Mace(One Handed)","Mace(Two Handed)"))); |
||||||
|
|
||||||
|
//SLOT 2 |
||||||
|
UltimateBag.EnableSlot2 = Config.Bind<bool>("3. WEAPON SLOT 2", "Enable Weapon Slot 2", true, "Add a weapon on your bag"); |
||||||
|
UltimateBag.VisualSlot2 = Config.Bind<int>("3. WEAPON SLOT 2", "Weapon ID", 2100999, "ItemID of the weapon you want to attach"); |
||||||
|
UltimateBag.PositionSlot2 = Config.Bind<string>("3. WEAPON SLOT 2", "Weapon Type", "Sword", new ConfigDescription("Choose the type of weapon select in Slot1. Required for positionning.", new AcceptableValueList<string>("Bow", "Spear", "Staff", "Axe(One Handed)", "Axe(Two Handed)", "Halberd", "Sword(One Handed)", "Sword(Two Handed)", "Mace(One Handed)", "Mace(Two Handed)"))); |
||||||
|
|
||||||
|
//SHIELD |
||||||
|
|
||||||
|
UltimateBag.EnableShield = Config.Bind<bool>("4. SHIELD", "Enable Shield", false, "Replace the backpack by a shield"); |
||||||
|
UltimateBag.ShieldVisual = Config.Bind<int>("4. SHIELD", "Shield ID", 2300050, "ItemID of the shield you want to attach"); |
||||||
|
|
||||||
|
//BOLT |
||||||
|
UltimateBag.AddBoltBool = Config.Bind<bool>("5.EFFECT", "Add Bolt Effect", true, "Add a bolt effect on your bag"); |
||||||
|
UltimateBag.BoltColor = Config.Bind<string>("5.EFFECT", "Color Bolt Effect", "yellow", new ConfigDescription("Choose color of bolt effect on your bag", new AcceptableValueList<string>("blue", "cyan", "gray", "green", "magenta", "red", "white", "yellow"))); |
||||||
|
|
||||||
|
//SPIRAL |
||||||
|
UltimateBag.AddSpiralBool = Config.Bind<bool>("6.EFFECT", "Add Spiral Effect", true, "Add a spiral effect on your bag"); |
||||||
|
UltimateBag.SpiralColor = Config.Bind<string>("6.EFFECT", "Color Spiral Effect", "yellow", new ConfigDescription("Choose color of spiral effect on your bag", new AcceptableValueList<string>("blue", "cyan", "gray", "green", "magenta", "red", "white", "yellow"))); |
||||||
|
|
||||||
|
//LANTERN |
||||||
|
UltimateBag.AddLanternBool = Config.Bind<bool>("7.LANTERN", "Add Lantern", true, "Add a bolt effect on your bag"); //BEPINEX CONFIGURATION MENU |
||||||
|
|
||||||
|
} |
||||||
|
|
||||||
|
void Update() { |
||||||
|
} //if (Input.GetKeyDown(KeyCode.N)) { //FOR TESTING BagSlot.Instance.Logger.LogMessage("KeyPress");}} // TESTING BLOCK |
||||||
|
|
||||||
|
static void ProcessVisualsExtend(Bag bag) |
||||||
|
{ |
||||||
|
|
||||||
|
if (bag.m_visualPrefabName == UltimateBag.BagPrefabName.Value) |
||||||
|
{ |
||||||
|
|
||||||
|
/* |
||||||
|
if (UltimateBag.BagPrefabName.Value == "5380005_BrigandBackpack_v") // DONE |
||||||
|
{ |
||||||
|
if (UltimateBag.AddLanternBool.Value) |
||||||
|
AddLanternSlot(bag, 0.0686f, 0.1271f, -0.2781f); //LanternSlot |
||||||
|
if (UltimateBag.AddBoltBool.Value) |
||||||
|
AddBolt(bag, 0f, 0.2f, 0f, UltimateBag.BoltColor.Value); // Bolt Effect |
||||||
|
if (UltimateBag.AddSpiralBool.Value) |
||||||
|
AddSpiral(bag, 0f, 0.2f, 0f, UltimateBag.SpiralColor.Value); // Spiral Effect |
||||||
|
if (UltimateBag.EnableSlot1.Value) |
||||||
|
AddWeaponSlot1(bag, VerifItemID(UltimateBag.VisualSlot1.Value, bow: true), -0.14f, 0.14f, 0f, 61.4246f, 141.3963f, 11.9091f); // Bow Attach |
||||||
|
//AddBow(bag, VerifItemID(UltimateBag.VisualSlot1.Value, bow: true), -0.14f, 0.14f, 0f, 61.4246f, 141.3963f, 11.9091f); |
||||||
|
if (UltimateBag.EnableSlot2.Value) |
||||||
|
AddWeaponSlot1(bag, VerifItemID(UltimateBag.VisualSlot2.Value, twoh: true), -0.28f, -0.035f, 0.48f, 2.734f, 64.5905f, 180.2475f); //2 Handed Sword Attach -0.28, -0.035, 0.48 |
||||||
|
} |
||||||
|
*/ |
||||||
|
|
||||||
|
// INITIALIZATION VARIABLE |
||||||
|
Vector3 positionSlot1 = new Vector3(-0.2101f, -0.0955f, -0.68f); |
||||||
|
Vector3 rotationSlot1 = new Vector3(1.0208f, 290.0177f, 179.9582f); |
||||||
|
Vector3 positionSlot2 = new Vector3(0.3f, -0.005f, -0.6f); |
||||||
|
Vector3 rotationSlot2 = new Vector3(357.5089f, 61.8f, 2.906f); |
||||||
|
string defineposandrot = UltimateBag.PositionSlot1.Value; |
||||||
|
string defineposandrot2 = UltimateBag.PositionSlot2.Value; |
||||||
|
|
||||||
|
// SWITCH FOR POSITION AND ROTATION |
||||||
|
switch (defineposandrot) //DONE |
||||||
|
{ |
||||||
|
case "Staff": |
||||||
|
positionSlot1 = new Vector3(-0.2101f, -0.0355f, -0.68f); |
||||||
|
rotationSlot1 = new Vector3(1.0208f, 290.0177f, 179.9582f); |
||||||
|
break; |
||||||
|
case "Bow": |
||||||
|
if (UltimateBag.BagPrefabName.Value == "5380005_BrigandBackpack_v") // SPECIAL PLACEMENT FOR BRIGANDBACKPACK |
||||||
|
{ |
||||||
|
positionSlot1 = new Vector3(-0.14f, 0.14f, 0f); |
||||||
|
rotationSlot1 = new Vector3(61.4246f, 141.3963f, 11.9091f); |
||||||
|
} else { |
||||||
|
positionSlot1 = new Vector3(0.04f, -0.04f, -0.14f); |
||||||
|
rotationSlot1 = new Vector3(356.3531f, 290.784f, 353.7287f); |
||||||
|
} |
||||||
|
break; |
||||||
|
case "Spear": |
||||||
|
positionSlot1 = new Vector3(-0.2901f, -0.0555f, -1f); |
||||||
|
rotationSlot1 = new Vector3(1.0208f, 290.0177f, 179.9582f); |
||||||
|
break; |
||||||
|
case "Axe(One Handed)": |
||||||
|
positionSlot1 = new Vector3(-0.02f, 0f, -0.4f); //-0.02, 0, -0.4 0.02f, -0.02f, -0.22f |
||||||
|
rotationSlot1 = new Vector3(5.2877f, 293.8563f, 175.003f); |
||||||
|
break; |
||||||
|
case "Axe(Two Handed)": |
||||||
|
positionSlot1 = new Vector3(0.22f, -0.095f, 0.24f); |
||||||
|
rotationSlot1 = new Vector3(2.8408f, 122.3997f, 188.2476f); |
||||||
|
break; |
||||||
|
case "Halberd": |
||||||
|
positionSlot1 = new Vector3(0.24f, -0.09f, 0.48f); |
||||||
|
rotationSlot1 = new Vector3(351.9301f, 292.5178f, 356.499f); |
||||||
|
break; |
||||||
|
case "Sword(One Handed)": |
||||||
|
positionSlot1 = new Vector3(-0.2413f, -0.031f, 0.2394f); //-0.02, 0, -0.4 0.02f, -0.02f, -0.22f |
||||||
|
rotationSlot1 = new Vector3(2.8648f, 59.127f, 179.9836f); |
||||||
|
break; |
||||||
|
case "Sword(Two Handed)": |
||||||
|
positionSlot1 = new Vector3(0.24f, -0.09f, 0.48f); |
||||||
|
rotationSlot1 = new Vector3(351.9301f, 292.5178f, 356.499f); |
||||||
|
break; |
||||||
|
case "Mace(One Handed)": |
||||||
|
positionSlot1 = new Vector3(-0.02f, 0f, -0.4f); //-0.02, 0, -0.4 0.02f, -0.02f, -0.22f |
||||||
|
rotationSlot1 = new Vector3(5.2877f, 293.8563f, 175.003f); |
||||||
|
break; |
||||||
|
case "Mace(Two Handed)": |
||||||
|
positionSlot1 = new Vector3(-0.2101f, -0.0355f, -0.68f); |
||||||
|
rotationSlot1 = new Vector3(1.0208f, 290.0177f, 179.9582f); |
||||||
|
break; |
||||||
|
default: |
||||||
|
break; |
||||||
|
} // POSITION AND ROTATION SLOT 1 |
||||||
|
|
||||||
|
switch (defineposandrot2) |
||||||
|
{ |
||||||
|
case "Staff": // DONE |
||||||
|
positionSlot2 = new Vector3(0.3f, -0.005f, -0.6f); |
||||||
|
rotationSlot2 = new Vector3(357.5089f, 61.8f, 2.906f); |
||||||
|
break; |
||||||
|
case "Bow": //DONE |
||||||
|
positionSlot2 = new Vector3(-0.04f, -0.04f, -0.12f); |
||||||
|
rotationSlot2 = new Vector3(2.734f, 64.5905f, 180.2475f); |
||||||
|
break; |
||||||
|
case "Spear": //DONE |
||||||
|
positionSlot2 = new Vector3(0.34f, -0.035f, -1.02f); |
||||||
|
rotationSlot2 = new Vector3(2.5851f, 250.0005f, 179.022f); |
||||||
|
break; |
||||||
|
case "Axe(One Handed)": |
||||||
|
positionSlot2 = new Vector3(0.1f, 0f, -0.4f); |
||||||
|
rotationSlot2 = new Vector3(358.5891f, 69.23f, 2.7964f); |
||||||
|
break; |
||||||
|
case "Axe(Two Handed)": |
||||||
|
positionSlot2 = new Vector3(-0.2f, 0.02f, 0.23f); |
||||||
|
rotationSlot2 = new Vector3(347.9792f, 231.782f, 6.1241f); |
||||||
|
break; |
||||||
|
case "Halberd": |
||||||
|
positionSlot2 = new Vector3(-0.26f, -0.07f, 0.51f); |
||||||
|
rotationSlot2 = new Vector3(8.9016f, 63.8621f, 181.4015f); |
||||||
|
break; |
||||||
|
case "Sword(One Handed)": |
||||||
|
positionSlot2 = new Vector3(0.28f, -0.08f, 0.24f); |
||||||
|
rotationSlot2 = new Vector3(359.7222f, 120.5592f, 184.8041f); |
||||||
|
break; |
||||||
|
case "Sword(Two Handed)": |
||||||
|
positionSlot2 = new Vector3(-0.28f, -0.035f, 0.48f); |
||||||
|
rotationSlot2 = new Vector3(2.734f, 64.5905f, 180.2475f); |
||||||
|
break; |
||||||
|
case "Mace(One Handed)": |
||||||
|
positionSlot2 = new Vector3(0.1f, 0f, -0.4f); |
||||||
|
rotationSlot2 = new Vector3(358.5891f, 69.23f, 2.7964f); |
||||||
|
break; |
||||||
|
case "Mace(Two Handed)": |
||||||
|
positionSlot2 = new Vector3(0.3f, -0.005f, -0.6f); |
||||||
|
rotationSlot2 = new Vector3(357.5089f, 61.8f, 2.906f); |
||||||
|
break; |
||||||
|
default: |
||||||
|
break; |
||||||
|
} // POSITION AND ROTATION SLOT 2 |
||||||
|
|
||||||
|
// POSITION AND ROTATION SHIELD |
||||||
|
Vector3 positionShield = new Vector3(0.0499f, 0.025f, -0.28f); |
||||||
|
Vector3 rotationShield = new Vector3(333.5798f, 282.9698f, 353.0449f); |
||||||
|
|
||||||
|
//PROCESS |
||||||
|
if (UltimateBag.AddBoltBool.Value) |
||||||
|
AddBolt(bag, 0f, 0.2f, 0f, UltimateBag.BoltColor.Value); // Bolt Effect |
||||||
|
if (UltimateBag.AddSpiralBool.Value) |
||||||
|
AddSpiral(bag, 0f, 0.2f, 0f, UltimateBag.SpiralColor.Value); // Spiral Effect |
||||||
|
if (UltimateBag.EnableSlot1.Value) |
||||||
|
AddWeaponSlot1(bag, VerifItemID(UltimateBag.VisualSlot1.Value, bow: true), positionSlot1, rotationSlot1); // Slot1 |
||||||
|
if (UltimateBag.EnableSlot2.Value) |
||||||
|
AddWeaponSlot2(bag, VerifItemID(UltimateBag.VisualSlot2.Value, twoh: true), positionSlot2, rotationSlot2); |
||||||
|
if (UltimateBag.AddLanternBool.Value || UltimateBag.BagPrefabName.Value == "5380005_BrigandBackpack_v") |
||||||
|
AddLanternSlot(bag, 0.0686f, 0.1271f, -0.2781f); //LanternSlot // PENSER A FAIRE UNE VALEUR POUR CHAQUE SAC SANS LANTERN |
||||||
|
if (UltimateBag.EnableShield.Value) |
||||||
|
AddShield(bag, VerifItemID(UltimateBag.ShieldVisual.Value), positionShield, rotationShield);// Slot2 |
||||||
|
|
||||||
|
|
||||||
|
//2300050 SHIELD WOLF |
||||||
|
} |
||||||
|
|
||||||
|
} |
||||||
|
|
||||||
|
|
||||||
|
|
||||||
|
static void AddLanternSlot(Bag bag, float xPos, float yPos, float zPos) |
||||||
|
{ |
||||||
|
|
||||||
|
Bag adventurerBackpack = (Bag)ResourcesPrefabManager.ITEM_PREFABS["5300000"]; |
||||||
|
|
||||||
|
Transform lanternHolder = adventurerBackpack.LoadedVisual.transform.Find("LanternSlotAnchor"); // FIND LANTERN ANCHOR |
||||||
|
|
||||||
|
Transform cachedLanternAnchor = Instantiate(lanternHolder); // CLONE ANCHOR |
||||||
|
|
||||||
|
cachedLanternAnchor.parent = bag.LoadedVisual.transform; |
||||||
|
|
||||||
|
bag.m_lanternSlot = bag.GetComponentInChildren<BagSlotVisual>(); |
||||||
|
|
||||||
|
cachedLanternAnchor.localPosition = new Vector3(xPos, yPos, zPos); //0.0686f, 0.1271f, -0.2781f |
||||||
|
|
||||||
|
} |
||||||
|
|
||||||
|
/* |
||||||
|
static void AddWeaponSlot2(Bag bag, int itemID, float xPos, float yPos, float zPos, float xRot, float yRot, float zRot) |
||||||
|
{ |
||||||
|
Vector3 roteuler = new Vector3(xRot, yRot, zRot); |
||||||
|
|
||||||
|
GameObject clone2HW = Instantiate(GetVisuals(itemID)); // Clone |
||||||
|
|
||||||
|
clone2HW.transform.parent = bag.LoadedVisual.transform; |
||||||
|
|
||||||
|
clone2HW.transform.localPosition = new Vector3(xPos, yPos, zPos);// Position |
||||||
|
|
||||||
|
clone2HW.transform.localRotation = Quaternion.Euler(roteuler); //Rotation /!\ REQUIRE EULER/NORMALIZER VALUES |
||||||
|
|
||||||
|
clone2HW.transform.parent = bag.LoadedVisual.transform; // Attach |
||||||
|
} |
||||||
|
*/ |
||||||
|
|
||||||
|
|
||||||
|
|
||||||
|
static void AddWeaponSlot2(Bag bag, int itemID, Vector3 Pos, Vector3 Rot) |
||||||
|
{ |
||||||
|
Vector3 rot = Rot; // Assignation |
||||||
|
|
||||||
|
GameObject cloneSlot2 = Instantiate(GetVisuals(itemID)); // Clone |
||||||
|
|
||||||
|
cloneSlot2.transform.parent = bag.LoadedVisual.transform; // Attach |
||||||
|
|
||||||
|
cloneSlot2.transform.localPosition = Pos; // Position |
||||||
|
|
||||||
|
cloneSlot2.transform.localRotation = Quaternion.Euler(rot); // Rotation |
||||||
|
} |
||||||
|
|
||||||
|
static void AddWeaponSlot1(Bag bag, int itemID, Vector3 Pos, Vector3 Rot) |
||||||
|
{ |
||||||
|
Vector3 rot = Rot; // Assignation |
||||||
|
|
||||||
|
GameObject cloneSlot1 = Instantiate(GetVisuals(itemID)); // Clone |
||||||
|
|
||||||
|
cloneSlot1.transform.parent = bag.LoadedVisual.transform; // Attach |
||||||
|
|
||||||
|
cloneSlot1.transform.localPosition = Pos; // Position |
||||||
|
|
||||||
|
cloneSlot1.transform.localRotation = Quaternion.Euler(rot); //Rotation /!\ REQUIRE EULER/NORMALIZER VALUES |
||||||
|
|
||||||
|
|
||||||
|
} |
||||||
|
|
||||||
|
static void AddShield(Bag bag, int itemID, Vector3 Pos, Vector3 Rot) |
||||||
|
{ |
||||||
|
|
||||||
|
Vector3 rot = Rot; // Assignation |
||||||
|
|
||||||
|
GameObject shield = Instantiate(GetVisuals(itemID)); // Clone |
||||||
|
|
||||||
|
shield.transform.parent = bag.LoadedVisual.transform; // Attach |
||||||
|
|
||||||
|
shield.transform.localPosition = Pos; // Position |
||||||
|
|
||||||
|
shield.transform.localRotation = Quaternion.Euler(rot); // Rotation |
||||||
|
|
||||||
|
Transform model = bag.LoadedVisual.transform.Find("model"); |
||||||
|
|
||||||
|
model.gameObject.SetActive(false); |
||||||
|
|
||||||
|
} |
||||||
|
|
||||||
|
static void AddBolt(Bag bag, float x, float y, float z, string color = "yellow", bool hidelight = false) |
||||||
|
{ |
||||||
|
|
||||||
|
Bag mageBackpack = (Bag)ResourcesPrefabManager.ITEM_PREFABS["5300170"]; |
||||||
|
|
||||||
|
Transform bolt = mageBackpack.LoadedVisual.transform.Find("backpack").transform.Find("_boltCrackling_Example_FX"); |
||||||
|
|
||||||
|
Transform cachedBolt = Instantiate(bolt.transform); |
||||||
|
|
||||||
|
cachedBolt.parent = bag.LoadedVisual.transform; |
||||||
|
|
||||||
|
cachedBolt.localPosition = new Vector3(x, y, z); |
||||||
|
|
||||||
|
if (hidelight == true) |
||||||
|
|
||||||
|
{ |
||||||
|
cachedBolt.transform.GetComponentInChildren<Light>().intensity = 0; |
||||||
|
|
||||||
|
} |
||||||
|
|
||||||
|
Color colorchoice = ToColor(color); |
||||||
|
|
||||||
|
cachedBolt.transform.GetComponentInChildren<ParticleSystem>().startColor = colorchoice; |
||||||
|
|
||||||
|
cachedBolt.transform.GetComponentInChildren<Light>().color = colorchoice; |
||||||
|
|
||||||
|
cachedBolt.parent = bag.LoadedVisual.transform; |
||||||
|
} |
||||||
|
|
||||||
|
static void AddSpiral(Bag bag, float x, float y, float z, string color = "yellow") |
||||||
|
{ |
||||||
|
Bag glowBackpack = (Bag)ResourcesPrefabManager.ITEM_PREFABS["5300050"]; |
||||||
|
|
||||||
|
Transform spiral = glowBackpack.LoadedVisual.transform.Find("Particle System"); |
||||||
|
|
||||||
|
Transform cachedSpiral = Instantiate(spiral.transform); |
||||||
|
|
||||||
|
cachedSpiral.parent = bag.LoadedVisual.transform; |
||||||
|
|
||||||
|
cachedSpiral.localPosition = new Vector3(x, y, z); |
||||||
|
|
||||||
|
Color colorchoice = ToColor(color); |
||||||
|
|
||||||
|
cachedSpiral.transform.GetComponentInChildren<ParticleSystem>().startColor = colorchoice; |
||||||
|
|
||||||
|
cachedSpiral.parent = bag.LoadedVisual.transform; |
||||||
|
|
||||||
|
} |
||||||
|
|
||||||
|
|
||||||
|
//////////// UTILITY /////////////// |
||||||
|
|
||||||
|
public static int VerifItemID(int itemid, bool bag = false, bool bow = false, bool twoh = false) |
||||||
|
{ |
||||||
|
if (ResourcesPrefabManager.ITEM_PREFABS[itemid.ToString()].name != null) |
||||||
|
{ |
||||||
|
return itemid; |
||||||
|
} |
||||||
|
else |
||||||
|
{ |
||||||
|
if (bag == true) |
||||||
|
{ |
||||||
|
return 5300000; |
||||||
|
} |
||||||
|
else if (bow == true) |
||||||
|
{ |
||||||
|
return 2200000; |
||||||
|
} |
||||||
|
else if (twoh == true) |
||||||
|
{ |
||||||
|
return 2100999; |
||||||
|
} |
||||||
|
else return 5300000; |
||||||
|
|
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
public static Color ToColor(string color) |
||||||
|
{ |
||||||
|
return (Color)typeof(Color).GetProperty(color.ToLowerInvariant()).GetValue(null, null); |
||||||
|
} |
||||||
|
|
||||||
|
public static GameObject GetVisuals(int itemID) |
||||||
|
{ |
||||||
|
string visualsPath = ResourcesPrefabManager.ITEM_PREFABS[itemID.ToString()].m_visualPrefabPath; |
||||||
|
return ResourcesPrefabManager.Instance.m_itemVisualsBundle.LoadAsset<GameObject>(visualsPath + ".prefab"); |
||||||
|
} |
||||||
|
|
||||||
|
|
||||||
|
//////////// PATCH /////////////// |
||||||
|
|
||||||
|
[HarmonyPatch(typeof(Bag), "BaseInit"), HarmonyPrefix] |
||||||
|
static void Bag_BaseInit_Pre(Bag __instance) |
||||||
|
{ |
||||||
|
ProcessVisualsExtend(__instance); |
||||||
|
} |
||||||
|
|
||||||
|
//////////// CONFIG ///////////// |
||||||
|
|
||||||
|
public static ConfigEntry<string> PositionSlot1; |
||||||
|
public static ConfigEntry<string> PositionSlot2; |
||||||
|
public static ConfigEntry<bool> EnableSlot1; |
||||||
|
public static ConfigEntry<bool> EnableSlot2; |
||||||
|
public static ConfigEntry<bool> AddBoltBool; |
||||||
|
public static ConfigEntry<string> BoltColor; |
||||||
|
public static ConfigEntry<bool> AddSpiralBool; |
||||||
|
public static ConfigEntry<string> SpiralColor; |
||||||
|
public static ConfigEntry<bool> AddLanternBool; |
||||||
|
public static ConfigEntry<string> BagPrefabName; |
||||||
|
public static ConfigEntry<int> VisualSlot1; |
||||||
|
public static ConfigEntry<int> VisualSlot2; |
||||||
|
|
||||||
|
public static ConfigEntry<bool> EnableShield; |
||||||
|
public static ConfigEntry<int> ShieldVisual; |
||||||
|
|
||||||
|
public static AcceptableValueList<string> CurrentBag; |
||||||
|
|
||||||
|
public static ConfigEntry<bool> isStaffS1; |
||||||
|
public static ConfigEntry<bool> isStaffS2; |
||||||
|
public static ConfigEntry<bool> isSpearS1; |
||||||
|
public static ConfigEntry<bool> isSpearS2; |
||||||
|
public static ConfigEntry<bool> isBowS1; |
||||||
|
public static ConfigEntry<bool> isBowS2; |
||||||
|
public static ConfigEntry<bool> isSwordS1; |
||||||
|
public static ConfigEntry<bool> isSwordS2; |
||||||
|
public static ConfigEntry<bool> isHalberdS1; //CONFIG ENTRY FOR BEPINEX CONFIGURATION MANAGER |
||||||
|
|
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
|
||||||
|
|
||||||
|
|
||||||
|
|
@ -0,0 +1,47 @@ |
|||||||
|
# Ultimate backpack |
||||||
|
by Ness |
||||||
|
|
||||||
|
> [NEXUS MOD MANAGER LINK](https://www.nexusmods.com/outward/mods/229) |
||||||
|
|
||||||
|
Ultimate backpack ****is simple mod for adding weapons, shield, lantern(for unplanned bags) and effects on your backpack. (PURELY COSMECTIC) |
||||||
|
|
||||||
|
*Disclaimer: it's my first mod, and i never used C# before, so... You can encounter some bugs. Try to play with the parameters, and be award that any mods don't touch visual of backpack.* |
||||||
|
|
||||||
|
If you want to help, you can send me your log [location: C:\Users\[USERNAME]\AppData\LocalLow\Nine Dots Studio\Outward]) |
||||||
|
|
||||||
|
## Require: |
||||||
|
[BepinEx Configuration Manager](https://github.com/BepInEx/BepInEx.ConfigurationManager) |
||||||
|
|
||||||
|
## How To Install: |
||||||
|
|
||||||
|
1. If you haven't already, download and install **BepInEx**: [instructions here](https://outward.gamepedia.com/Installing_Mods#BepInEx_Loader) |
||||||
|
2. Download the **Ultimatebackpack.zip** file from the Files page |
||||||
|
3. Put this zip file in your Outward directory, so it looks like "**Outward\**Ultimatebackpack**.zip**" |
||||||
|
4. Right-click the file and **Extract Here**, so it merges with the folders. It should look like "Outward\BepInEx\plugins\UltimateBackpack\" |
||||||
|
5. Done! (you can delete the .zip file if you want) |
||||||
|
|
||||||
|
## How to configure: |
||||||
|
|
||||||
|
1. Press F1 (or F5) in game to open the Configuration Manager |
||||||
|
2. Select your current backpack equipped |
||||||
|
3. Enable weapon slot 1 or/and weapon slot 2 |
||||||
|
4. Put the item id of the weapon you want to attach on your backpack |
||||||
|
[WEAPON ID HERE](https://outward.fandom.com/wiki/Equipment#Weapons) |
||||||
|
|
||||||
|
7. Select the type of each weapon you want to attach |
||||||
|
|
||||||
|
9. Enjoy! |
||||||
|
10. (Optionnal) You can replace the backpack visual by a shield, for that, just select the shield id you want to attach and enable replace on configuration manager |
||||||
|
|
||||||
|
12. (Optionnal) Add effects and color on your bag + lantern slot if your bag don't have one |
||||||
|
|
||||||
|
**Thanks To** |
||||||
|
|
||||||
|
- [Sinai](https://www.nexusmods.com/outward/users/68319717?tab=user+files) and [Vheos](https://www.nexusmods.com/outward/users/58085611?tab=user+files) for their help and advice in the outward-modding channel |
||||||
|
|
||||||
|
### GALLERY: |
||||||
|
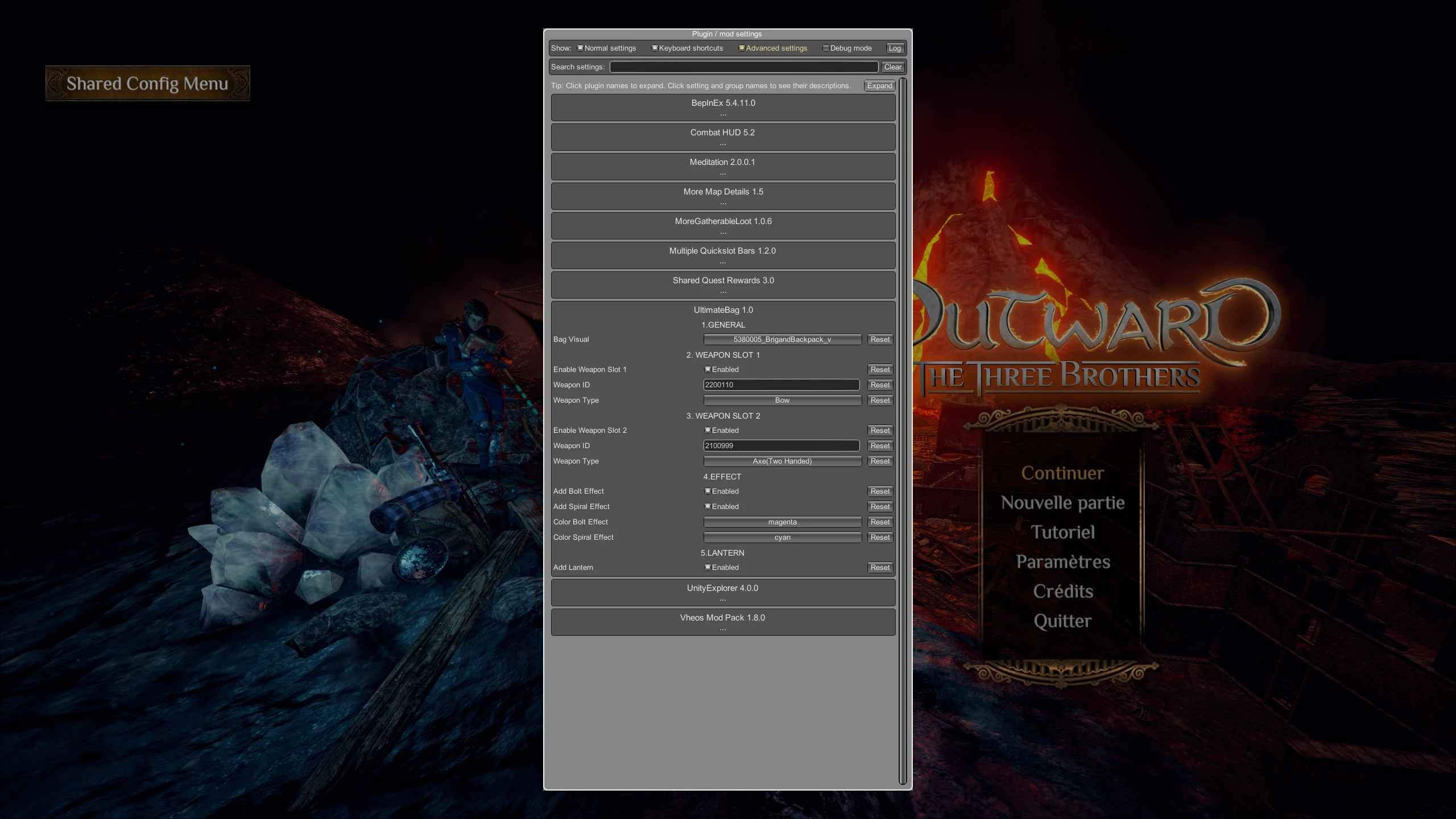 |
||||||
|
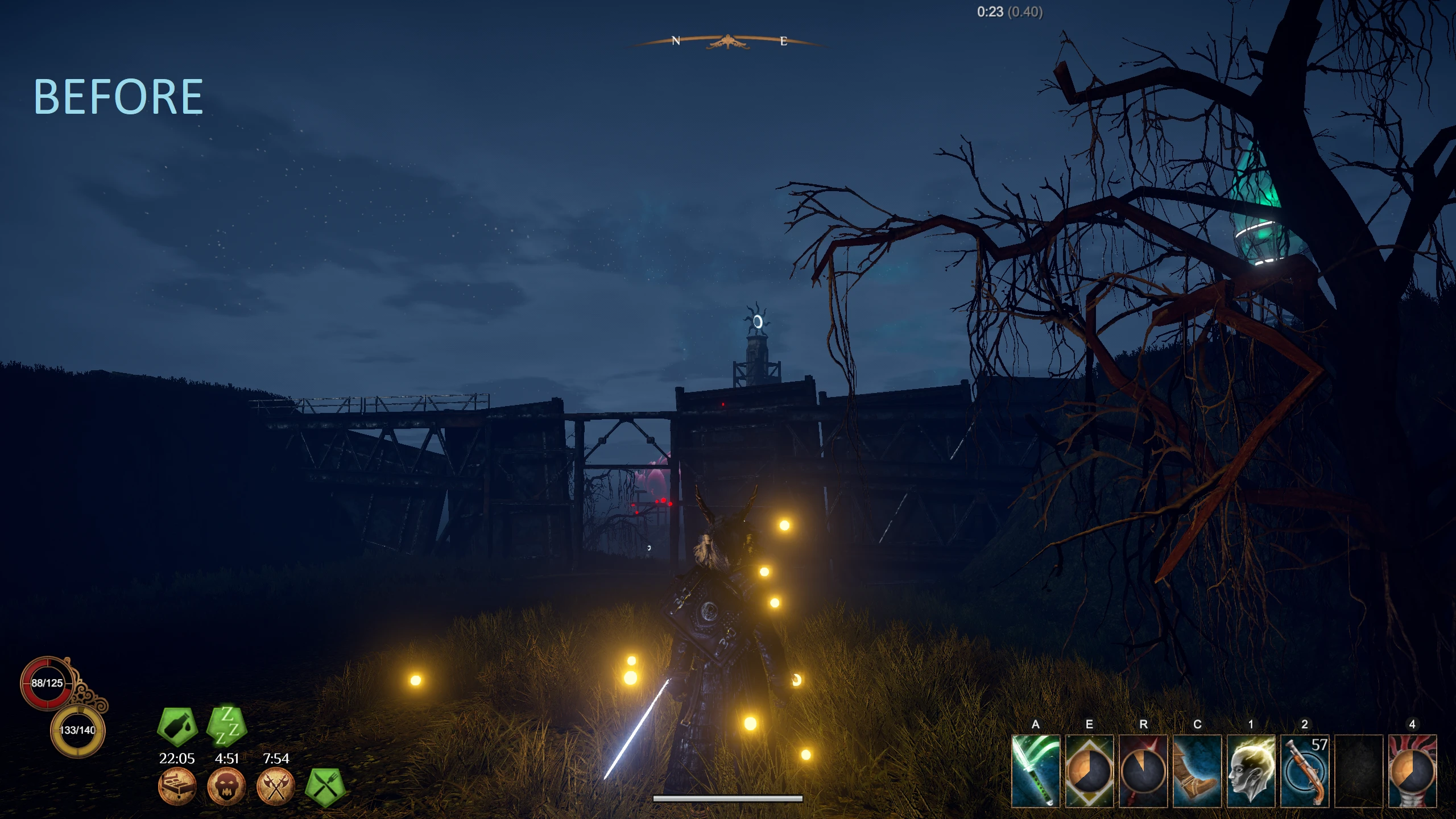 |
||||||
|
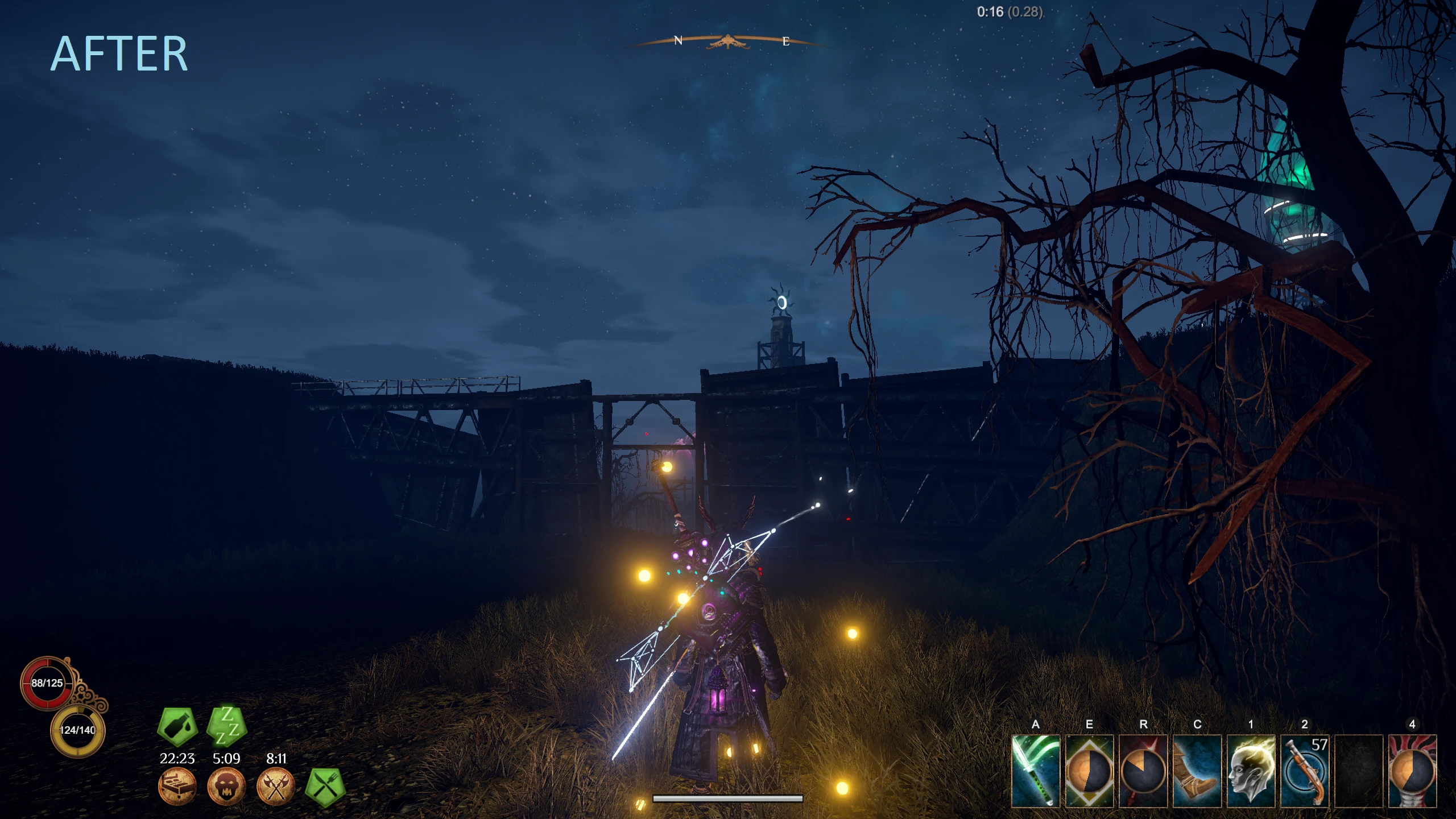 |
||||||
|
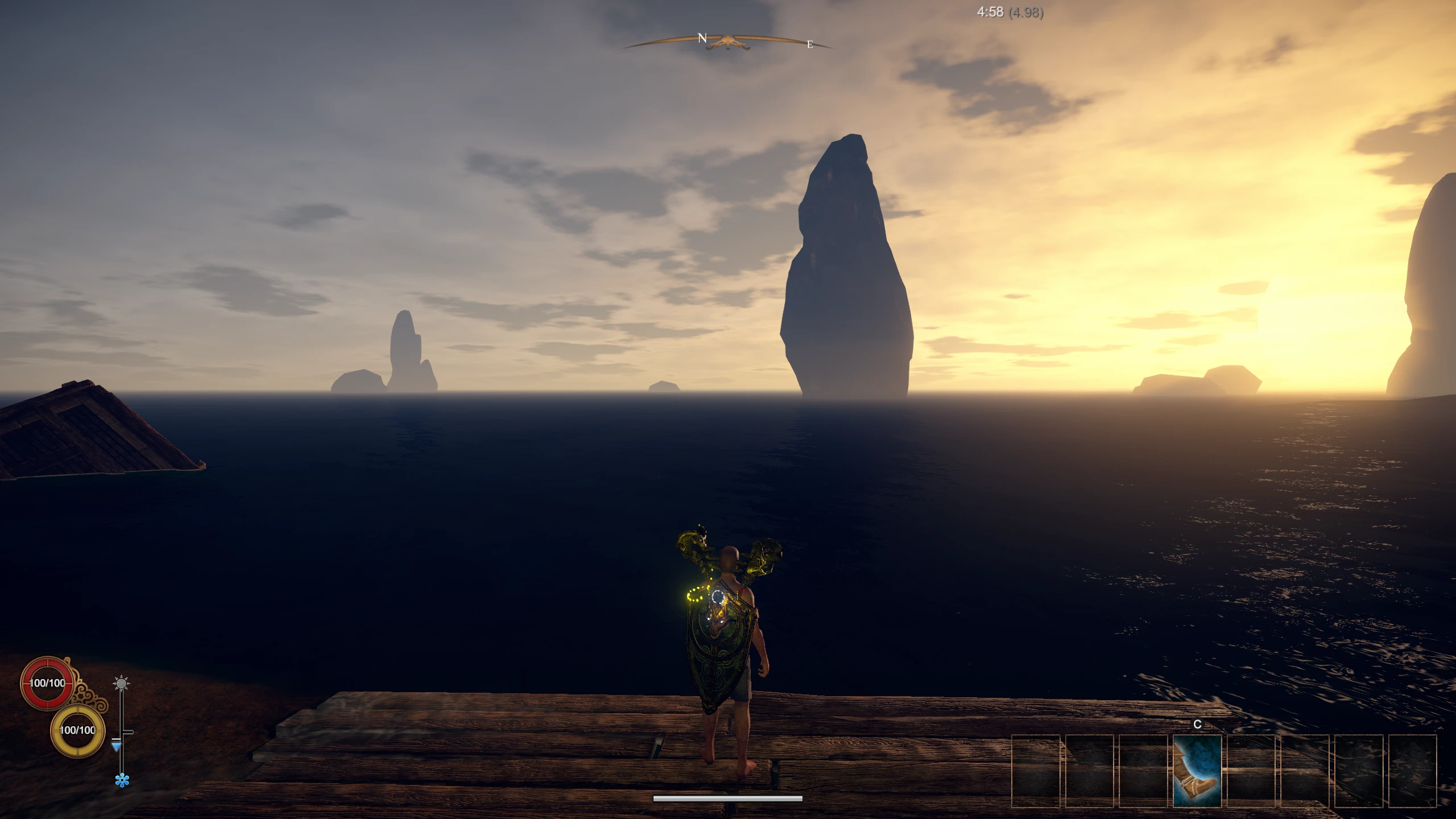 |
||||||
|
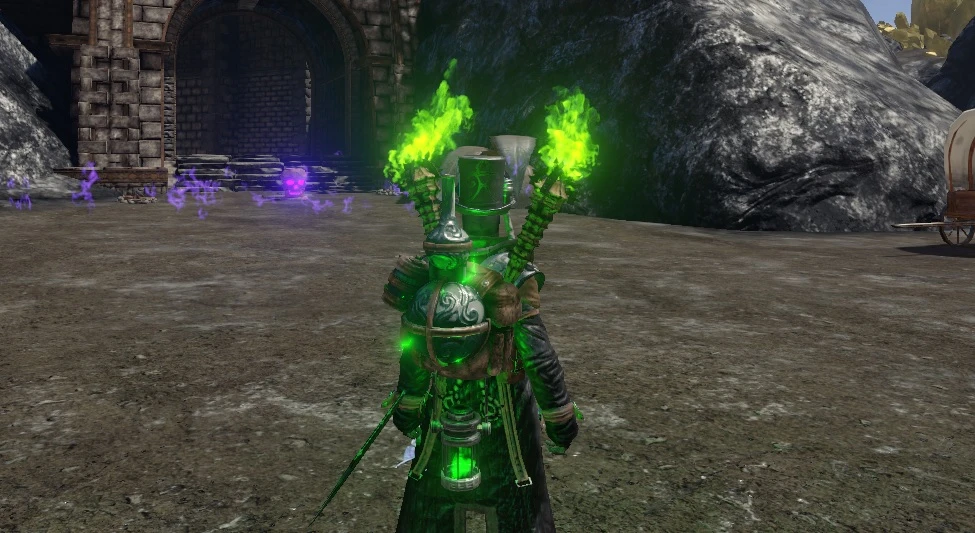 |
@ -0,0 +1,85 @@ |
|||||||
|
<?xml version="1.0" encoding="utf-8"?> |
||||||
|
<Project ToolsVersion="15.0" xmlns="http://schemas.microsoft.com/developer/msbuild/2003"> |
||||||
|
<Import Project="$(MSBuildExtensionsPath)\$(MSBuildToolsVersion)\Microsoft.Common.props" Condition="Exists('$(MSBuildExtensionsPath)\$(MSBuildToolsVersion)\Microsoft.Common.props')" /> |
||||||
|
<PropertyGroup> |
||||||
|
<Configuration Condition=" '$(Configuration)' == '' ">Debug</Configuration> |
||||||
|
<Platform Condition=" '$(Platform)' == '' ">AnyCPU</Platform> |
||||||
|
<ProjectGuid>{70034B0D-05EA-41E6-A276-46BCA3101217}</ProjectGuid> |
||||||
|
<OutputType>Library</OutputType> |
||||||
|
<AppDesignerFolder>Properties</AppDesignerFolder> |
||||||
|
<RootNamespace>UltimateBag</RootNamespace> |
||||||
|
<AssemblyName>UltimateBag</AssemblyName> |
||||||
|
<TargetFrameworkVersion>v4.7.2</TargetFrameworkVersion> |
||||||
|
<FileAlignment>512</FileAlignment> |
||||||
|
<Deterministic>true</Deterministic> |
||||||
|
</PropertyGroup> |
||||||
|
<PropertyGroup Condition=" '$(Configuration)|$(Platform)' == 'Debug|AnyCPU' "> |
||||||
|
<DebugSymbols>true</DebugSymbols> |
||||||
|
<DebugType>full</DebugType> |
||||||
|
<Optimize>false</Optimize> |
||||||
|
<OutputPath>bin\Debug\</OutputPath> |
||||||
|
<DefineConstants>DEBUG;TRACE</DefineConstants> |
||||||
|
<ErrorReport>prompt</ErrorReport> |
||||||
|
<WarningLevel>4</WarningLevel> |
||||||
|
<AllowUnsafeBlocks>true</AllowUnsafeBlocks> |
||||||
|
</PropertyGroup> |
||||||
|
<PropertyGroup Condition=" '$(Configuration)|$(Platform)' == 'Release|AnyCPU' "> |
||||||
|
<DebugType>pdbonly</DebugType> |
||||||
|
<Optimize>true</Optimize> |
||||||
|
<OutputPath>bin\Release\</OutputPath> |
||||||
|
<DefineConstants>TRACE</DefineConstants> |
||||||
|
<ErrorReport>prompt</ErrorReport> |
||||||
|
<WarningLevel>4</WarningLevel> |
||||||
|
<AllowUnsafeBlocks>true</AllowUnsafeBlocks> |
||||||
|
</PropertyGroup> |
||||||
|
<ItemGroup> |
||||||
|
<Reference Include="0Harmony"> |
||||||
|
<HintPath>J:\Games\SteamLibrary\steamapps\common\Outward\BepInEx\core\0Harmony.dll</HintPath> |
||||||
|
</Reference> |
||||||
|
<Reference Include="Assembly-CSharp-firstpass_publicized"> |
||||||
|
<HintPath>J:\Games\SteamLibrary\steamapps\common\Outward\Outward_Data\Managed\publicized_assemblies\Assembly-CSharp-firstpass_publicized.dll</HintPath> |
||||||
|
</Reference> |
||||||
|
<Reference Include="Assembly-CSharp_publicized"> |
||||||
|
<HintPath>J:\Games\SteamLibrary\steamapps\common\Outward\Outward_Data\Managed\publicized_assemblies\Assembly-CSharp_publicized.dll</HintPath> |
||||||
|
</Reference> |
||||||
|
<Reference Include="BepInEx"> |
||||||
|
<HintPath>J:\Games\SteamLibrary\steamapps\common\Outward\BepInEx\core\BepInEx.dll</HintPath> |
||||||
|
</Reference> |
||||||
|
<Reference Include="BepInEx.MessageCenter"> |
||||||
|
<HintPath>J:\Games\SteamLibrary\steamapps\common\Outward\BepInEx\plugins\BepInEx.MessageCenter.dll</HintPath> |
||||||
|
</Reference> |
||||||
|
<Reference Include="ConfigurationManager"> |
||||||
|
<HintPath>J:\Games\SteamLibrary\steamapps\common\Outward\BepInEx\plugins\ConfigurationManager.dll</HintPath> |
||||||
|
</Reference> |
||||||
|
<Reference Include="System" /> |
||||||
|
<Reference Include="System.Core" /> |
||||||
|
<Reference Include="System.Xml.Linq" /> |
||||||
|
<Reference Include="System.Data.DataSetExtensions" /> |
||||||
|
<Reference Include="Microsoft.CSharp" /> |
||||||
|
<Reference Include="System.Data" /> |
||||||
|
<Reference Include="System.Net.Http" /> |
||||||
|
<Reference Include="System.Xml" /> |
||||||
|
<Reference Include="UnityEngine"> |
||||||
|
<HintPath>J:\Games\SteamLibrary\steamapps\common\Outward\Outward_Data\Managed\UnityEngine.dll</HintPath> |
||||||
|
</Reference> |
||||||
|
<Reference Include="UnityEngine.AssetBundleModule, Version=0.0.0.0, Culture=neutral, PublicKeyToken=null" /> |
||||||
|
<Reference Include="UnityEngine.CoreModule, Version=0.0.0.0, Culture=neutral, processorArchitecture=MSIL"> |
||||||
|
<SpecificVersion>False</SpecificVersion> |
||||||
|
<HintPath>J:\Games\SteamLibrary\steamapps\common\Outward\Outward_Data\Managed\UnityEngine.CoreModule.dll</HintPath> |
||||||
|
</Reference> |
||||||
|
<Reference Include="UnityEngine.IMGUIModule, Version=0.0.0.0, Culture=neutral, processorArchitecture=MSIL"> |
||||||
|
<SpecificVersion>False</SpecificVersion> |
||||||
|
<HintPath>J:\Games\SteamLibrary\steamapps\common\Outward\Outward_Data\Managed\UnityEngine.IMGUIModule.dll</HintPath> |
||||||
|
</Reference> |
||||||
|
<Reference Include="UnityEngine.ParticleSystemModule, Version=0.0.0.0, Culture=neutral, processorArchitecture=MSIL"> |
||||||
|
<SpecificVersion>False</SpecificVersion> |
||||||
|
<HintPath>J:\Games\SteamLibrary\steamapps\common\Outward\Outward_Data\Managed\UnityEngine.ParticleSystemModule.dll</HintPath> |
||||||
|
</Reference> |
||||||
|
</ItemGroup> |
||||||
|
<ItemGroup> |
||||||
|
<Compile Include="ConfigurationManagerAttributes.cs" /> |
||||||
|
<Compile Include="Main.cs" /> |
||||||
|
<Compile Include="Properties\AssemblyInfo.cs" /> |
||||||
|
</ItemGroup> |
||||||
|
<Import Project="$(MSBuildToolsPath)\Microsoft.CSharp.targets" /> |
||||||
|
</Project> |
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
@ -0,0 +1,298 @@ |
|||||||
|
<?xml version="1.0"?> |
||||||
|
<doc> |
||||||
|
<assembly> |
||||||
|
<name>ConfigurationManager</name> |
||||||
|
</assembly> |
||||||
|
<members> |
||||||
|
<member name="M:ConfigurationManager.LegacySettingSearcher.GetLegacyPluginConfig(BepInEx.BaseUnityPlugin)"> |
||||||
|
<summary> |
||||||
|
Used by bepinex 4 plugins |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="M:ConfigurationManager.SettingSearcher.GetBepInExCoreConfig"> |
||||||
|
<summary> |
||||||
|
Bepinex 5 config |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="M:ConfigurationManager.SettingSearcher.GetPluginConfig(BepInEx.BaseUnityPlugin)"> |
||||||
|
<summary> |
||||||
|
Used by bepinex 5 plugins |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="M:ConfigurationManager.Utilities.Utils.FilterBrowsable``1(System.Collections.Generic.IEnumerable{``0},System.Boolean,System.Boolean)"> |
||||||
|
<summary> |
||||||
|
Return items with browsable attribute same as expectedBrowsable, and optionally items with no browsable attribute |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="T:ConfigurationManager.ConfigurationManager"> |
||||||
|
<summary> |
||||||
|
An easy way to let user configure how a plugin behaves without the need to make your own GUI. The user can change any of the settings you expose, even keyboard shortcuts. |
||||||
|
https://github.com/ManlyMarco/BepInEx.ConfigurationManager |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="F:ConfigurationManager.ConfigurationManager.GUID"> |
||||||
|
<summary> |
||||||
|
GUID of this plugin |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="F:ConfigurationManager.ConfigurationManager.Version"> |
||||||
|
<summary> |
||||||
|
Version constant |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="E:ConfigurationManager.ConfigurationManager.DisplayingWindowChanged"> |
||||||
|
<summary> |
||||||
|
Event fired every time the manager window is shown or hidden. |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="F:ConfigurationManager.ConfigurationManager.OverrideHotkey"> |
||||||
|
<summary> |
||||||
|
Disable the hotkey check used by config manager. If enabled you have to set <see cref="P:ConfigurationManager.ConfigurationManager.DisplayingWindow"/> to show the manager. |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="M:ConfigurationManager.ConfigurationManager.#ctor"> |
||||||
|
<inheritdoc /> |
||||||
|
</member> |
||||||
|
<member name="P:ConfigurationManager.ConfigurationManager.DisplayingWindow"> |
||||||
|
<summary> |
||||||
|
Is the config manager main window displayed on screen |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="M:ConfigurationManager.ConfigurationManager.RegisterCustomSettingDrawer(System.Type,System.Action{ConfigurationManager.SettingEntryBase})"> |
||||||
|
<summary> |
||||||
|
Register a custom setting drawer for a given type. The action is ran in OnGui in a single setting slot. |
||||||
|
Do not use any Begin / End layout methods, and avoid raising height from standard. |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="P:ConfigurationManager.ConfigurationManager.SearchString"> |
||||||
|
<summary> |
||||||
|
String currently entered into the search box |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="P:ConfigurationManager.LegacySettingEntry.Wrapper"> |
||||||
|
<summary> |
||||||
|
Instance of the object that holds this setting. |
||||||
|
Null if setting is not in a ConfigWrapper. |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="T:ConfigurationManager.SettingEntryBase"> |
||||||
|
<summary> |
||||||
|
Class representing all data about a setting collected by ConfigurationManager. |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="P:ConfigurationManager.SettingEntryBase.AcceptableValues"> |
||||||
|
<summary> |
||||||
|
List of values this setting can take |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="P:ConfigurationManager.SettingEntryBase.AcceptableValueRange"> |
||||||
|
<summary> |
||||||
|
Range of the values this setting can take |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="P:ConfigurationManager.SettingEntryBase.ShowRangeAsPercent"> |
||||||
|
<summary> |
||||||
|
Should the setting be shown as a percentage (only applies to value range settings) |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="P:ConfigurationManager.SettingEntryBase.CustomDrawer"> |
||||||
|
<summary> |
||||||
|
Custom setting draw action |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="P:ConfigurationManager.SettingEntryBase.Browsable"> |
||||||
|
<summary> |
||||||
|
Show this setting in the settings screen at all? If false, don't show. |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="P:ConfigurationManager.SettingEntryBase.Category"> |
||||||
|
<summary> |
||||||
|
Category the setting is under. Null to be directly under the plugin. |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="P:ConfigurationManager.SettingEntryBase.DefaultValue"> |
||||||
|
<summary> |
||||||
|
If set, a "Default" button will be shown next to the setting to allow resetting to default. |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="P:ConfigurationManager.SettingEntryBase.HideDefaultButton"> |
||||||
|
<summary> |
||||||
|
Force the "Reset" button to not be displayed, even if a valid DefaultValue is available. |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="P:ConfigurationManager.SettingEntryBase.HideSettingName"> |
||||||
|
<summary> |
||||||
|
Force the setting name to not be displayed. Should only be used with a <see cref="P:ConfigurationManager.SettingEntryBase.CustomDrawer"/> to get more space. |
||||||
|
Can be used together with <see cref="P:ConfigurationManager.SettingEntryBase.HideDefaultButton"/> to gain even more space. |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="P:ConfigurationManager.SettingEntryBase.Description"> |
||||||
|
<summary> |
||||||
|
Optional description shown when hovering over the setting |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="P:ConfigurationManager.SettingEntryBase.DispName"> |
||||||
|
<summary> |
||||||
|
Name of the setting |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="P:ConfigurationManager.SettingEntryBase.PluginInfo"> |
||||||
|
<summary> |
||||||
|
Plugin this setting belongs to |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="P:ConfigurationManager.SettingEntryBase.ReadOnly"> |
||||||
|
<summary> |
||||||
|
Only allow showing of the value. False whenever possible by default. |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="P:ConfigurationManager.SettingEntryBase.SettingType"> |
||||||
|
<summary> |
||||||
|
Type of the variable |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="P:ConfigurationManager.SettingEntryBase.PluginInstance"> |
||||||
|
<summary> |
||||||
|
Instance of the plugin that owns this setting |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="P:ConfigurationManager.SettingEntryBase.IsAdvanced"> |
||||||
|
<summary> |
||||||
|
Is this setting advanced |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="P:ConfigurationManager.SettingEntryBase.Order"> |
||||||
|
<summary> |
||||||
|
Order of the setting on the settings list relative to other settings in a category. 0 by default, lower is higher on the list. |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="M:ConfigurationManager.SettingEntryBase.Get"> |
||||||
|
<summary> |
||||||
|
Get the value of this setting |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="M:ConfigurationManager.SettingEntryBase.Set(System.Object)"> |
||||||
|
<summary> |
||||||
|
Set the value of this setting |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="M:ConfigurationManager.SettingEntryBase.SetValue(System.Object)"> |
||||||
|
<summary> |
||||||
|
Implementation of <see cref="M:ConfigurationManager.SettingEntryBase.Set(System.Object)"/> |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="P:ConfigurationManager.SettingEntryBase.ObjToStr"> |
||||||
|
<summary> |
||||||
|
Custom converter from setting type to string for the textbox |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="P:ConfigurationManager.SettingEntryBase.StrToObj"> |
||||||
|
<summary> |
||||||
|
Custom converter from string to setting type for the textbox |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="T:ConfigurationManager.ValueChangedEventArgs`1"> |
||||||
|
<summary> |
||||||
|
Arguments representing a change in value |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="M:ConfigurationManager.ValueChangedEventArgs`1.#ctor(`0)"> |
||||||
|
<inheritdoc /> |
||||||
|
</member> |
||||||
|
<member name="P:ConfigurationManager.ValueChangedEventArgs`1.NewValue"> |
||||||
|
<summary> |
||||||
|
Newly assigned value |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="T:BepInEx.AcceptableValueListAttribute"> |
||||||
|
<summary> |
||||||
|
Specify the list of acceptable values for this variable. It will allow the configuration window to show a list of available values. |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="M:BepInEx.AcceptableValueListAttribute.#ctor(System.Object[])"> |
||||||
|
<summary> |
||||||
|
Specify the list of acceptable values for this variable. It will allow the configuration window to show a list of available values. |
||||||
|
</summary> |
||||||
|
<param name="acceptableValues">List of acceptable values for this setting</param> |
||||||
|
</member> |
||||||
|
<member name="M:BepInEx.AcceptableValueListAttribute.#ctor(System.String)"> |
||||||
|
<summary> |
||||||
|
Specify a method that returns the list of acceptable values for this variable. It will allow the configuration window to show a list of available values. |
||||||
|
</summary> |
||||||
|
<param name="acceptableValueGetterName">Name of an instance method that takes no arguments and returns array object[] that contains the acceptable values</param> |
||||||
|
</member> |
||||||
|
<member name="T:BepInEx.AcceptableValueRangeAttribute"> |
||||||
|
<summary> |
||||||
|
Specify the range of acceptable values for this variable. It will allow the configuration window to show a slider |
||||||
|
and filter inputs. |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="M:BepInEx.AcceptableValueRangeAttribute.#ctor(System.Object,System.Object,System.Boolean)"> |
||||||
|
<param name="minValue">Lowest acceptable value</param> |
||||||
|
<param name="maxValue">Highest acceptable value</param> |
||||||
|
<param name="showAsPercentage"> |
||||||
|
Show the current value as % between min and max values if possible. Otherwise show the |
||||||
|
value itself. |
||||||
|
</param> |
||||||
|
</member> |
||||||
|
<member name="M:BepInEx.CustomSettingDrawAttribute.#ctor(System.String)"> |
||||||
|
<summary> |
||||||
|
Register a custom field editor drawer that will replace config manager's default field editors |
||||||
|
(The part between setting name and the default button). |
||||||
|
</summary> |
||||||
|
<param name="customFieldDrawMethod">Name of the method that will draw the edit field. |
||||||
|
The method needs to be an instance method with signature <code>void Name ()</code>. Runs in OnGUI.</param> |
||||||
|
</member> |
||||||
|
<member name="T:BepInEx.KeyboardShortcut"> |
||||||
|
<summary> |
||||||
|
A keyboard shortcut that can be used in Update method to check if user presses a key combo. |
||||||
|
Use SavedKeyboardShortcut to allow user to change this shortcut and have the changes saved. |
||||||
|
How to use: Use IsDown instead of the Imput.GetKeyDown in the Update loop. |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="M:BepInEx.KeyboardShortcut.#ctor(UnityEngine.KeyCode,UnityEngine.KeyCode[])"> |
||||||
|
<summary> |
||||||
|
Create a new keyboard shortcut. |
||||||
|
</summary> |
||||||
|
<param name="mainKey">Main key to press</param> |
||||||
|
<param name="modifiers">Keys that should be held down before main key is registered</param> |
||||||
|
</member> |
||||||
|
<member name="M:BepInEx.KeyboardShortcut.IsDown"> |
||||||
|
<summary> |
||||||
|
Check if the main key was just pressed (Input.GetKeyDown), and specified modifier keys are all pressed |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="M:BepInEx.KeyboardShortcut.IsPressed"> |
||||||
|
<summary> |
||||||
|
Check if the main key is currently held down (Input.GetKey), and specified modifier keys are all pressed |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="M:BepInEx.KeyboardShortcut.IsUp"> |
||||||
|
<summary> |
||||||
|
Check if the main key was just lifted (Input.GetKeyUp), and specified modifier keys are all pressed. |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="T:BepInEx.SavedKeyboardShortcut"> |
||||||
|
<summary> |
||||||
|
A keyboard shortcut that is saved in the config file and can be changed by the user if ConfigurationManager plugin |
||||||
|
is present. |
||||||
|
How to use: Run IsPressed in Update to check if user presses the button combo. |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="M:BepInEx.SavedKeyboardShortcut.IsPressed"> |
||||||
|
<summary> |
||||||
|
Check if the main key is currently held down (Input.GetKey), and specified modifier keys are all pressed |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="M:BepInEx.SavedKeyboardShortcut.IsDown"> |
||||||
|
<summary> |
||||||
|
Check if the main key was just pressed (Input.GetKeyDown), and specified modifier keys are all pressed |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="M:BepInEx.SavedKeyboardShortcut.IsUp"> |
||||||
|
<summary> |
||||||
|
Check if the main key was just lifted (Input.GetKeyUp), and specified modifier keys are all pressed. |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
</members> |
||||||
|
</doc> |
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
@ -0,0 +1,178 @@ |
|||||||
|
<?xml version="1.0"?> |
||||||
|
<doc> |
||||||
|
<assembly> |
||||||
|
<name>MonoMod.RuntimeDetour</name> |
||||||
|
</assembly> |
||||||
|
<members> |
||||||
|
<member name="T:MonoMod.RuntimeDetour.Detour"> |
||||||
|
<summary> |
||||||
|
A fully managed detour. |
||||||
|
Multiple Detours for a method to detour from can exist at any given time. Detours can be layered. |
||||||
|
If you're writing your own detour manager or need to detour native functions, it's better to create instances of NativeDetour instead. |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="M:MonoMod.RuntimeDetour.Detour.Apply"> |
||||||
|
<summary> |
||||||
|
Mark the detour as applied in the detour chain. This can be done automatically when creating an instance. |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="M:MonoMod.RuntimeDetour.Detour.Undo"> |
||||||
|
<summary> |
||||||
|
Undo the detour without freeing it, allowing you to reapply it later. |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="M:MonoMod.RuntimeDetour.Detour.Free"> |
||||||
|
<summary> |
||||||
|
Free the detour, while also permanently undoing it. This makes any further operations on this detour invalid. |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="M:MonoMod.RuntimeDetour.Detour.Dispose"> |
||||||
|
<summary> |
||||||
|
Undo and free this temporary detour. |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="M:MonoMod.RuntimeDetour.Detour.GenerateTrampoline(System.Reflection.MethodBase)"> |
||||||
|
<summary> |
||||||
|
Generate a new DynamicMethod with which you can invoke the previous state. |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="M:MonoMod.RuntimeDetour.Detour.GenerateTrampoline``1"> |
||||||
|
<summary> |
||||||
|
Generate a new DynamicMethod with which you can invoke the previous state. |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="M:MonoMod.RuntimeDetour.Hook.GenerateTrampoline``1"> |
||||||
|
<summary> |
||||||
|
Generate a new DynamicMethod with which you can invoke the previous state. |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="T:MonoMod.RuntimeDetour.NativeDetour"> |
||||||
|
<summary> |
||||||
|
A "raw" native detour, acting as a wrapper around NativeDetourData with a few helpers. |
||||||
|
Only one NativeDetour for a method to detour from can exist at any given time. NativeDetours cannot be layered. |
||||||
|
If you don't need the trampoline generator or any of the management helpers, use DetourManager.Native directly. |
||||||
|
Unless you're writing your own detour manager or need to detour native functions, it's better to create instances of Detour instead. |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="M:MonoMod.RuntimeDetour.NativeDetour.Apply"> |
||||||
|
<summary> |
||||||
|
Apply the native detour. This can be done automatically when creating an instance. |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="M:MonoMod.RuntimeDetour.NativeDetour.Undo"> |
||||||
|
<summary> |
||||||
|
Undo the native detour without freeing the detour native data, allowing you to reapply it later. |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="M:MonoMod.RuntimeDetour.NativeDetour.ChangeSource(System.IntPtr)"> |
||||||
|
<summary> |
||||||
|
Changes the source of this native detour to a new source address. This does not repair the old source location. |
||||||
|
This also assumes that <paramref name="newSource"/> is simply a new address for the same method as this was constructed with. |
||||||
|
</summary> |
||||||
|
<param name="newSource">The new source location.</param> |
||||||
|
</member> |
||||||
|
<member name="M:MonoMod.RuntimeDetour.NativeDetour.ChangeTarget(System.IntPtr)"> |
||||||
|
<summary> |
||||||
|
Changed the target of this native detour to a new target. |
||||||
|
</summary> |
||||||
|
<param name="newTarget">The new target address.</param> |
||||||
|
</member> |
||||||
|
<member name="M:MonoMod.RuntimeDetour.NativeDetour.Free"> |
||||||
|
<summary> |
||||||
|
Free the detour's data without undoing it. This makes any further operations on this detour invalid. |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="M:MonoMod.RuntimeDetour.NativeDetour.Dispose"> |
||||||
|
<summary> |
||||||
|
Undo and free this temporary detour. |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="M:MonoMod.RuntimeDetour.NativeDetour.GenerateTrampoline(System.Reflection.MethodBase)"> |
||||||
|
<summary> |
||||||
|
Generate a new DynamicMethod with which you can invoke the previous state. |
||||||
|
If the NativeDetour holds a reference to a managed method, a copy of the original method is returned. |
||||||
|
If the NativeDetour holds a reference to a native function, an "undo-call-redo" trampoline with a matching signature is returned. |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="M:MonoMod.RuntimeDetour.NativeDetour.GenerateTrampoline``1"> |
||||||
|
<summary> |
||||||
|
Generate a new delegate with which you can invoke the previous state. |
||||||
|
If the NativeDetour holds a reference to a managed method, a copy of the original method is returned. |
||||||
|
If the NativeDetour holds a reference to a native function, an "undo-call-redo" trampoline with a matching signature is returned. |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="M:MonoMod.RuntimeDetour.DetourHelper.Write(System.IntPtr,System.Int32@,System.Byte)"> |
||||||
|
<summary> |
||||||
|
Write the given value at the address to + offs, afterwards advancing offs by sizeof(byte). |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="M:MonoMod.RuntimeDetour.DetourHelper.Write(System.IntPtr,System.Int32@,System.UInt16)"> |
||||||
|
<summary> |
||||||
|
Write the given value at the address to + offs, afterwards advancing offs by sizeof(ushort). |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="M:MonoMod.RuntimeDetour.DetourHelper.Write(System.IntPtr,System.Int32@,System.UInt32)"> |
||||||
|
<summary> |
||||||
|
Write the given value at the address to + offs, afterwards advancing offs by sizeof(ushort). |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="M:MonoMod.RuntimeDetour.DetourHelper.Write(System.IntPtr,System.Int32@,System.UInt64)"> |
||||||
|
<summary> |
||||||
|
Write the given value at the address to + offs, afterwards advancing offs by sizeof(ulong). |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="M:MonoMod.RuntimeDetour.DetourHelper.GenerateNativeProxy(System.IntPtr,System.Reflection.MethodBase)"> |
||||||
|
<summary> |
||||||
|
Generate a DynamicMethod to easily call the given native function from another DynamicMethod. |
||||||
|
</summary> |
||||||
|
<param name="target">The pointer to the native function to call.</param> |
||||||
|
<param name="signature">A MethodBase with the target function's signature.</param> |
||||||
|
<returns>The detoured DynamicMethod.</returns> |
||||||
|
</member> |
||||||
|
<member name="M:MonoMod.RuntimeDetour.DetourHelper.StubCriticalDetour(MonoMod.Utils.DynamicMethodDefinition)"> |
||||||
|
<summary> |
||||||
|
Fill the DynamicMethodDefinition with a throw. |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="M:MonoMod.RuntimeDetour.DetourHelper.EmitDetourCopy(Mono.Cecil.Cil.ILProcessor,System.IntPtr,System.IntPtr,System.Byte)"> |
||||||
|
<summary> |
||||||
|
Emit a call to DetourManager.Native.Copy using the given parameters. |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="M:MonoMod.RuntimeDetour.DetourHelper.EmitDetourApply(Mono.Cecil.Cil.ILProcessor,MonoMod.RuntimeDetour.NativeDetourData)"> |
||||||
|
<summary> |
||||||
|
Emit a call to DetourManager.Native.Apply using a copy of the given data. |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="T:MonoMod.RuntimeDetour.NativeDetourData"> |
||||||
|
<summary> |
||||||
|
The data forming a "raw" native detour, created and consumed by DetourManager.Native. |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="F:MonoMod.RuntimeDetour.NativeDetourData.Method"> |
||||||
|
<summary> |
||||||
|
The method to detour from. Set when the structure is created by the IDetourNativePlatform. |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="F:MonoMod.RuntimeDetour.NativeDetourData.Target"> |
||||||
|
<summary> |
||||||
|
The target method to be called instead. Set when the structure is created by the IDetourNativePlatform. |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="F:MonoMod.RuntimeDetour.NativeDetourData.Type"> |
||||||
|
<summary> |
||||||
|
The type of the detour. Determined when the structure is created by the IDetourNativePlatform. |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="F:MonoMod.RuntimeDetour.NativeDetourData.Size"> |
||||||
|
<summary> |
||||||
|
The size of the detour. Calculated when the structure is created by the IDetourNativePlatform. |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="F:MonoMod.RuntimeDetour.NativeDetourData.Extra"> |
||||||
|
<summary> |
||||||
|
DetourManager.Native-specific data. |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
</members> |
||||||
|
</doc> |
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
@ -0,0 +1,178 @@ |
|||||||
|
<?xml version="1.0"?> |
||||||
|
<doc> |
||||||
|
<assembly> |
||||||
|
<name>MonoMod.RuntimeDetour</name> |
||||||
|
</assembly> |
||||||
|
<members> |
||||||
|
<member name="T:MonoMod.RuntimeDetour.Detour"> |
||||||
|
<summary> |
||||||
|
A fully managed detour. |
||||||
|
Multiple Detours for a method to detour from can exist at any given time. Detours can be layered. |
||||||
|
If you're writing your own detour manager or need to detour native functions, it's better to create instances of NativeDetour instead. |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="M:MonoMod.RuntimeDetour.Detour.Apply"> |
||||||
|
<summary> |
||||||
|
Mark the detour as applied in the detour chain. This can be done automatically when creating an instance. |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="M:MonoMod.RuntimeDetour.Detour.Undo"> |
||||||
|
<summary> |
||||||
|
Undo the detour without freeing it, allowing you to reapply it later. |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="M:MonoMod.RuntimeDetour.Detour.Free"> |
||||||
|
<summary> |
||||||
|
Free the detour, while also permanently undoing it. This makes any further operations on this detour invalid. |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="M:MonoMod.RuntimeDetour.Detour.Dispose"> |
||||||
|
<summary> |
||||||
|
Undo and free this temporary detour. |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="M:MonoMod.RuntimeDetour.Detour.GenerateTrampoline(System.Reflection.MethodBase)"> |
||||||
|
<summary> |
||||||
|
Generate a new DynamicMethod with which you can invoke the previous state. |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="M:MonoMod.RuntimeDetour.Detour.GenerateTrampoline``1"> |
||||||
|
<summary> |
||||||
|
Generate a new DynamicMethod with which you can invoke the previous state. |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="M:MonoMod.RuntimeDetour.Hook.GenerateTrampoline``1"> |
||||||
|
<summary> |
||||||
|
Generate a new DynamicMethod with which you can invoke the previous state. |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="T:MonoMod.RuntimeDetour.NativeDetour"> |
||||||
|
<summary> |
||||||
|
A "raw" native detour, acting as a wrapper around NativeDetourData with a few helpers. |
||||||
|
Only one NativeDetour for a method to detour from can exist at any given time. NativeDetours cannot be layered. |
||||||
|
If you don't need the trampoline generator or any of the management helpers, use DetourManager.Native directly. |
||||||
|
Unless you're writing your own detour manager or need to detour native functions, it's better to create instances of Detour instead. |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="M:MonoMod.RuntimeDetour.NativeDetour.Apply"> |
||||||
|
<summary> |
||||||
|
Apply the native detour. This can be done automatically when creating an instance. |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="M:MonoMod.RuntimeDetour.NativeDetour.Undo"> |
||||||
|
<summary> |
||||||
|
Undo the native detour without freeing the detour native data, allowing you to reapply it later. |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="M:MonoMod.RuntimeDetour.NativeDetour.ChangeSource(System.IntPtr)"> |
||||||
|
<summary> |
||||||
|
Changes the source of this native detour to a new source address. This does not repair the old source location. |
||||||
|
This also assumes that <paramref name="newSource"/> is simply a new address for the same method as this was constructed with. |
||||||
|
</summary> |
||||||
|
<param name="newSource">The new source location.</param> |
||||||
|
</member> |
||||||
|
<member name="M:MonoMod.RuntimeDetour.NativeDetour.ChangeTarget(System.IntPtr)"> |
||||||
|
<summary> |
||||||
|
Changed the target of this native detour to a new target. |
||||||
|
</summary> |
||||||
|
<param name="newTarget">The new target address.</param> |
||||||
|
</member> |
||||||
|
<member name="M:MonoMod.RuntimeDetour.NativeDetour.Free"> |
||||||
|
<summary> |
||||||
|
Free the detour's data without undoing it. This makes any further operations on this detour invalid. |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="M:MonoMod.RuntimeDetour.NativeDetour.Dispose"> |
||||||
|
<summary> |
||||||
|
Undo and free this temporary detour. |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="M:MonoMod.RuntimeDetour.NativeDetour.GenerateTrampoline(System.Reflection.MethodBase)"> |
||||||
|
<summary> |
||||||
|
Generate a new DynamicMethod with which you can invoke the previous state. |
||||||
|
If the NativeDetour holds a reference to a managed method, a copy of the original method is returned. |
||||||
|
If the NativeDetour holds a reference to a native function, an "undo-call-redo" trampoline with a matching signature is returned. |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="M:MonoMod.RuntimeDetour.NativeDetour.GenerateTrampoline``1"> |
||||||
|
<summary> |
||||||
|
Generate a new delegate with which you can invoke the previous state. |
||||||
|
If the NativeDetour holds a reference to a managed method, a copy of the original method is returned. |
||||||
|
If the NativeDetour holds a reference to a native function, an "undo-call-redo" trampoline with a matching signature is returned. |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="M:MonoMod.RuntimeDetour.DetourHelper.Write(System.IntPtr,System.Int32@,System.Byte)"> |
||||||
|
<summary> |
||||||
|
Write the given value at the address to + offs, afterwards advancing offs by sizeof(byte). |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="M:MonoMod.RuntimeDetour.DetourHelper.Write(System.IntPtr,System.Int32@,System.UInt16)"> |
||||||
|
<summary> |
||||||
|
Write the given value at the address to + offs, afterwards advancing offs by sizeof(ushort). |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="M:MonoMod.RuntimeDetour.DetourHelper.Write(System.IntPtr,System.Int32@,System.UInt32)"> |
||||||
|
<summary> |
||||||
|
Write the given value at the address to + offs, afterwards advancing offs by sizeof(ushort). |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="M:MonoMod.RuntimeDetour.DetourHelper.Write(System.IntPtr,System.Int32@,System.UInt64)"> |
||||||
|
<summary> |
||||||
|
Write the given value at the address to + offs, afterwards advancing offs by sizeof(ulong). |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="M:MonoMod.RuntimeDetour.DetourHelper.GenerateNativeProxy(System.IntPtr,System.Reflection.MethodBase)"> |
||||||
|
<summary> |
||||||
|
Generate a DynamicMethod to easily call the given native function from another DynamicMethod. |
||||||
|
</summary> |
||||||
|
<param name="target">The pointer to the native function to call.</param> |
||||||
|
<param name="signature">A MethodBase with the target function's signature.</param> |
||||||
|
<returns>The detoured DynamicMethod.</returns> |
||||||
|
</member> |
||||||
|
<member name="M:MonoMod.RuntimeDetour.DetourHelper.StubCriticalDetour(MonoMod.Utils.DynamicMethodDefinition)"> |
||||||
|
<summary> |
||||||
|
Fill the DynamicMethodDefinition with a throw. |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="M:MonoMod.RuntimeDetour.DetourHelper.EmitDetourCopy(Mono.Cecil.Cil.ILProcessor,System.IntPtr,System.IntPtr,System.Byte)"> |
||||||
|
<summary> |
||||||
|
Emit a call to DetourManager.Native.Copy using the given parameters. |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="M:MonoMod.RuntimeDetour.DetourHelper.EmitDetourApply(Mono.Cecil.Cil.ILProcessor,MonoMod.RuntimeDetour.NativeDetourData)"> |
||||||
|
<summary> |
||||||
|
Emit a call to DetourManager.Native.Apply using a copy of the given data. |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="T:MonoMod.RuntimeDetour.NativeDetourData"> |
||||||
|
<summary> |
||||||
|
The data forming a "raw" native detour, created and consumed by DetourManager.Native. |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="F:MonoMod.RuntimeDetour.NativeDetourData.Method"> |
||||||
|
<summary> |
||||||
|
The method to detour from. Set when the structure is created by the IDetourNativePlatform. |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="F:MonoMod.RuntimeDetour.NativeDetourData.Target"> |
||||||
|
<summary> |
||||||
|
The target method to be called instead. Set when the structure is created by the IDetourNativePlatform. |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="F:MonoMod.RuntimeDetour.NativeDetourData.Type"> |
||||||
|
<summary> |
||||||
|
The type of the detour. Determined when the structure is created by the IDetourNativePlatform. |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="F:MonoMod.RuntimeDetour.NativeDetourData.Size"> |
||||||
|
<summary> |
||||||
|
The size of the detour. Calculated when the structure is created by the IDetourNativePlatform. |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
<member name="F:MonoMod.RuntimeDetour.NativeDetourData.Extra"> |
||||||
|
<summary> |
||||||
|
DetourManager.Native-specific data. |
||||||
|
</summary> |
||||||
|
</member> |
||||||
|
</members> |
||||||
|
</doc> |
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Some files were not shown because too many files have changed in this diff Show More
Loading…
Reference in new issue